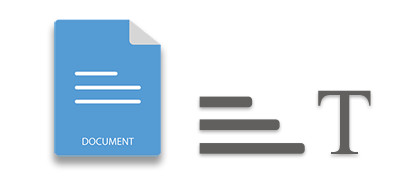
Microsoft Word documents play a crucial role in creating and sharing textual content. If you’re developing C# applications that need to interact with these documents, you may find yourself needing to extract text from Word documents using C# in ASP.NET while ensuring that formatting is preserved. Whether you’re analyzing text, extracting specific sections, or combining content, this guide will help you efficiently extract text from Word documents using the best C# library for word document text extraction.
Table of Contents
- C# Library to Extract Text from Word Documents
- Understanding Text Extraction in Word Documents
- Extracting Text from a Word Document
C# Library to Extract Text from Word Documents
Aspose.Words for .NET is a feature-rich, easy-to-use library designed for working with Word documents. It offers a comprehensive set of functionalities, including a .NET word text extraction API, document creation, manipulation, and conversion. Aspose.Words for .NET is an invaluable tool for developers seeking efficient C# word text extraction.
You can download the DLL or install the library directly from NuGet using the package manager console:
PM> Install-Package Aspose.Words
Understanding Text Extraction in Word Documents
An MS Word document is composed of various elements, such as paragraphs, tables, and images. Consequently, your text extraction requirements may differ based on your specific needs. For example, you might want to extract text from a scanned Word document in C# or extract text from a Word file using C# .NET. Each element in a Word document is represented as a node, which you’ll interact with during the extraction process. Let’s explore how to extract text from Word documents while effectively handling word formatting when extracting text in C#.
Extracting Text from a Word Document
In this section, we will implement a C# text extractor for Word documents. The workflow for text extraction includes:
- Defining the nodes to include in the text extraction process.
- Extracting content between the specified nodes (including or excluding the starting and ending nodes).
- Cloning the extracted nodes to create a new Word document containing the extracted content.
Let’s create a method named ExtractContent, which will accept the nodes and other parameters for text extraction. This method will parse the document and clone the nodes. Here are the parameters we’ll pass to the method:
- StartNode and EndNode serve as the starting and ending points for content extraction. These can be block-level (e.g., Paragraph, Table) or inline-level nodes (e.g., Run, FieldStart, BookmarkStart, etc.).
- For fields, pass the corresponding FieldStart object.
- For bookmarks, use BookmarkStart and BookmarkEnd nodes.
- For comments, utilize CommentRangeStart and CommentRangeEnd nodes.
- IsInclusive specifies whether the markers are included in the extraction. If set to false and the same or consecutive nodes are passed, an empty list will be returned.
The complete implementation of the ExtractContent method, which accurately extracts text from protected Word documents in C#, is as follows:
Additionally, some helper methods are required by the ExtractContent method to facilitate the text extraction operation:
Now we’re ready to utilize these methods and extract text from Word documents using C#.
Extracting Text between Paragraphs of a Word Document
To extract content between two paragraphs in a Word DOCX document, follow these steps:
- Load the Word document using the Document class.
- Reference the starting and ending paragraphs using the Document.FirstSection.Body.GetChild(NodeType.PARAGRAPH, int, boolean) method.
- Call the ExtractContent(startPara, endPara, true) method to extract the nodes into an object.
- Use the GenerateDocument(Document, extractedNodes) helper method to create a document containing the extracted content.
- Finally, save the returned document using the Document.Save(string) method.
Here’s a code sample demonstrating how to extract text from large Word files by extracting content between the 7th and 11th paragraphs:
Extracting Text between Different Types of Nodes
You can also extract content between different types of nodes. For example, let’s extract content between a paragraph and a table and save it into a new Word document. Follow these steps:
- Load the Word document using the Document class.
- Reference the starting and ending nodes using the Document.FirstSection.Body.GetChild(NodeType, int, boolean) method.
- Call the ExtractContent(startPara, endPara, true) method to extract the nodes into an object.
- Use the GenerateDocument(Document, extractedNodes) helper method to create a document containing the extracted content.
- Save the returned document using the Document.Save(string) method.
Here’s a code sample illustrating how to extract text between a paragraph and a table in C#:
Extracting Text between Paragraphs Based on Styles
Now let’s explore how to extract content between paragraphs based on styles. In this example, we will extract content between the first “Heading 1” and the first “Heading 3” in the Word document. Follow these steps:
- Load the Word document using the Document class.
- Extract paragraphs into an object using the ParagraphsByStyleName(Document, “Heading 1”) helper method.
- Extract paragraphs into another object using the ParagraphsByStyleName(Document, “Heading 3”) helper method.
- Call the ExtractContent(startPara, endPara, true) method, passing the first elements in both paragraph arrays as parameters.
- Use the GenerateDocument(Document, extractedNodes) helper method to create a document containing the extracted content.
- Finally, save the returned document using the Document.Save(string) method.
Here’s a code sample demonstrating how to extract content between paragraphs based on styles:
Read More about Text Extraction
Explore other scenarios of the .NET API for Word document text extraction in this documentation article.
Get Free Word Text Extractor Library
You can obtain a free temporary license to extract text without evaluation limitations.
Conclusion
Aspose.Words for .NET is a versatile library that streamlines the process of C# extracting text from Word while preserving formatting. With its extensive features and user-friendly API, you can efficiently work with Word documents and automate various scenarios involving handling special characters during C# word text extraction. Whether you’re developing applications that need to process Word documents or simply extracting text, Aspose.Words for .NET is a valuable tool for developers.
Explore additional features of Aspose.Words for .NET through the documentation. If you have any questions, feel free to reach out via our forum.
See Also
Tip: You might also want to check out the Aspose PowerPoint to Word Converter, which demonstrates the popular presentation-to-Word document conversion process.